Analyzing my workouts with the WHOOP API in Python
• • #python
In 2023 I did 279 workouts for a total duration of 169h 42m 2s, and burned 100.562 kcal. How do I know that? By using Python and the data from my WHOOP fitness band.
In this blog post I'll show you how you can do the same.
These are the full 2023 stats my little script is showing me:
❯ python analyze_workouts.py
Number of workouts in 2023: 279
Total kcal: 100562.94 kcal
Average kcal: 360.44 kcal
Total duration: 169h 42m 2s (7 days, 1:42:02)
Average duration: 0:36:29
Top 3 activities:
Running: 164
Functional Fitness: 58
Yoga: 42
How to work with the WHOOP API in Python
#The easiest way I've found to work with the WHOOP API is by using the https://github.com/hedgertronic/whoop package. This is a small Whoop API Client implementation that lets you easily work with the API and extract not only workout data but also other data collected with your WHOOP band.
This is how you can use it:
from whoop import WhoopClient
with WhoopClient(username, password) as client:
body_measurement = client.get_body_measurement()
workouts = client.get_workout_collection()
sleep_collection = client.get_sleep_collection()
recoveries = client.get_recovery_collection()
How to analyze WHOOP Workout data with Python
#I wrote a few Python scripts that utilize the whoop package to save all workouts into a JSON file and then extract a few statistics.
The full code is here: https://github.com/patrickloeber/whoop-analyzer
Clone the repo and follow the 3 steps below to use it.
Step 1: Installation
#pip install -r requirements.txt
The only dependencies listed in there are Authlib
and requests
.
Step 2: Get Workouts
#Set the following variables in get_workouts.py
:
username
: Your WHOOP email/usernamepassword
: Your WHOOP passwordstart_date
: The day until it should collect the workouts
Then run
python get_workouts.py
This will collect the workouts starting from today until the specified start date and dumps them into a file called workouts.json
.
Step 3: Analyze Workouts
#Run
python analyze_workouts.py
This loads the JSON file and prints the stats for 2023.
Note: The current logic filters for 2023 workouts and ignores activities categorized as Unknown, MISC, and Walking. If you want to modify this logic, change the code in the filter_workouts()
function.
How to identify the activity from the WHOOP sport_id
#WHOOP specifies a sport_id
for each workout. I compared these numbers with my workouts to identify the corresponding activity.
So far I've converted the following sport_id
values to workout names:
SPORT_ID_TO_NAME = {
0: "Running",
1: "Bicycle",
33: "Swimming",
96: "HIIT",
71: "MISC",
43: "Pilates",
44: "Yoga",
48: "Functional Fitness",
52: "Hiking",
-1: "Unknown Activity",
63: "Walking",
}
If you have a WHOOP band, too, and know other corresponding activities, feel free to create a pull request here.
Have fun with it!
Hope you enjoyed the read!
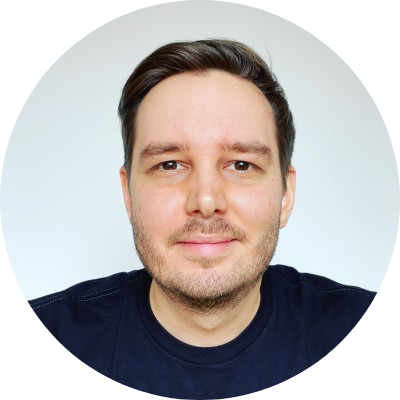
▶ Follow me on
Twitter
&
LinkedIn
▶ Tutorials on
YouTube
▶ Dev Advocate at
AssemblyAI
▶ Founder of
python-engineer.com